10 Severe PHP Vulnerabilities & How to Fix Them
PHP applications can be vulnerable to various hacking techniques if they have security vulnerabilities. Here are some common examples:
SQL Injection.
- Utilize parameterized queries or prepared statements to separate SQL code from user input.
- Sanitize and validate user input using functions like
mysqli_real_escape_string()
or use an ORM framework. - Implement input validation and strict data type checks.

Cross-Site Scripting (XSS).
- Perform output encoding or HTML entity encoding to prevent script execution.
- Use security libraries like HTML Purifier to sanitize user-generated content.
- Implement Content Security Policy (CSP) headers to mitigate XSS attacks.

Remote Code Execution (RCE).
- Avoid executing user-supplied input as system commands.
- Implement strict input validation and filtering.
- Use secure alternatives like
shell_exec()
instead of potentially unsafe functions likesystem()
.

File Inclusion.
- Avoid including files based on user input.
- Validate and sanitize user input before using it in the
include()
orrequire()
functions. - Implement a whitelist approach, allowing only predefined files to be included.
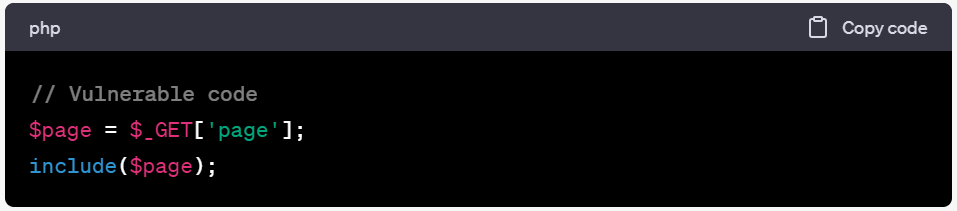
File Upload Vulnerabilities.
- Validate and verify uploaded files for file type, size, and content.
- Store uploaded files outside the web root directory.
- Implement file permissions to restrict access to uploaded files.
- Utilize PHP’s
move_uploaded_file()
function for secure file handling.
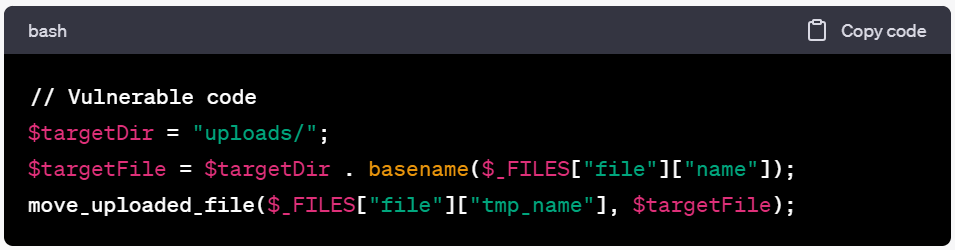
Cross-Site Request Forgery (CSRF):
- Implement CSRF tokens to validate and authenticate requests.
- Ensure that critical operations require explicit user consent, such as re-authentication or CAPTCHA verification.
- Set appropriate HTTP response headers to mitigate CSRF attacks.
Code Injection.
- Avoid using
eval()
or similar functions that execute dynamic code. - Implement input validation and strict data type checks.
- Apply proper escaping and filtering techniques when dealing with user-generated content.
Insecure Session Management.
- Use secure session management techniques, such as generating unique session IDs, setting session expiration, and using secure cookies.
- Implement session_regenerate_id() to prevent session fixation attacks.
- Store session data securely and avoid exposing sensitive information in session variables.
Remote File Inclusion (RFI).
- Avoid dynamically including files based on user input.
- Implement a whitelist approach for included files.
- Ensure that remote file inclusion is disabled or restricted in the server configuration.
Server Misconfigurations.
- Regularly update and patch PHP versions and related libraries to address known vulnerabilities.
- Harden server configurations, disable unnecessary PHP functions, and restrict file permissions.
- Regularly monitor and log server activities for suspicious behavior.
Implementing these security practices significantly reduces the risk of severe PHP vulnerabilities. It is important to keep up with the latest security advisories, follow secure coding guidelines, and conduct regular security assessments to ensure the ongoing protection of PHP applications.
Do you like to develop a new project? You can reach us anytime with a small brief, and we’ll get back to you shortly.